This is a boiler plate to get you started using DataStore.
If needed, use this official guide to do the initial Amplify setup.
The code below assumes you have the following GraphQl schema created which is similar to the example from the documentation link above.
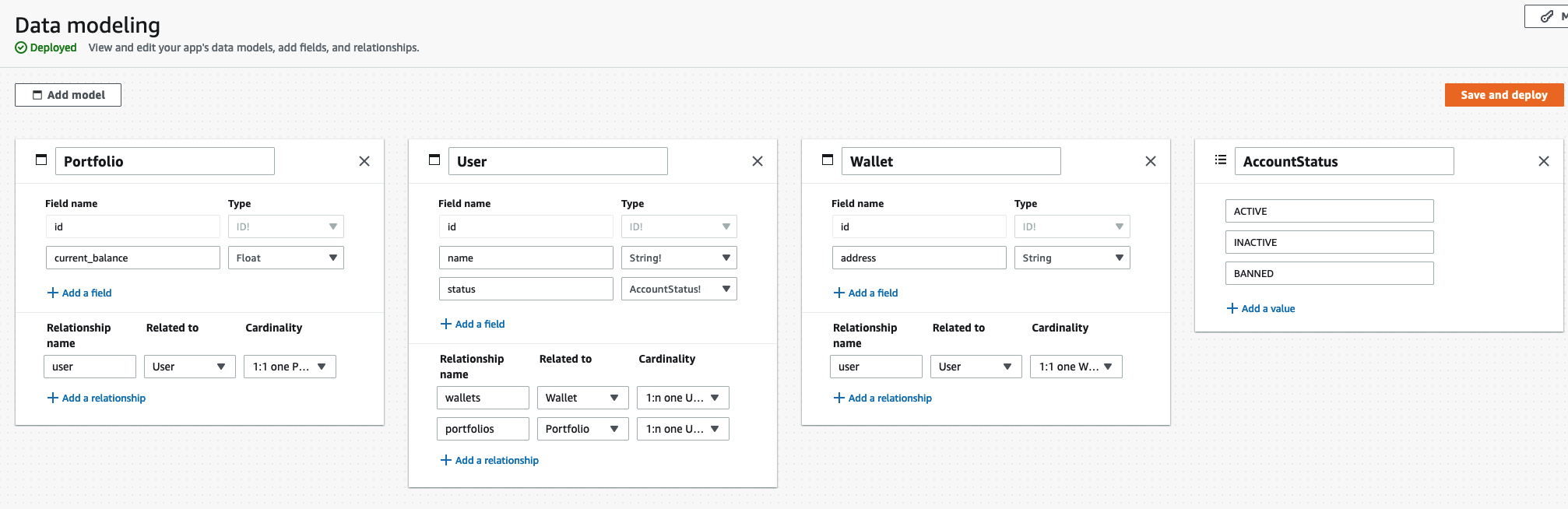
In this example I'm creating a one-many relationship between User and Portfolio/Wallet.
The models can be modifies in the file schema.graphql
by default located at amplify/backend/{api_name}/
. This is how it would look for this example:
type Portfolio @model {
id: ID!
current_balance: Float
user: User @connection(name: "UserPortfolio")
}
type User @model {
id: ID!
name: String!
status: AccountStatus!
wallets: [Wallet] @connection(name: "UserWallet")
portfolios: [Portfolio] @connection(name: "UserPortfolio")
}
type Wallet @model {
id: ID!
address: String
user: User @connection(name: "UserWallet")
}
enum AccountStatus {
ACTIVE
INACTIVE
BANNED
}
Write Data
VStack {
Button(action: {
let newUser = User(name: "Arturo", status: .active)
let newWallet = Wallet(address: "maWalleeeet", user: newUser)
Amplify.DataStore.save(newUser) { userResult in
switch userResult {
case .failure(let error):
print("Error adding user - \(error.localizedDescription)")
case .success:
Amplify.DataStore.save(newWallet) { walletResult in
switch walletResult {
case .success:
print("Wallet saved! \(walletResult)")
case .failure(let error):
print("Error adding wallet - \(error.localizedDescription)")
}
}
}
}
}) {
Text("Create User + Wallet")
}
}
Read Data
VStack {
Button(action: {
Amplify.DataStore.query(User.self) { result in
switch result {
case .success(let users):
let userId = users[0].id
Amplify.DataStore.query(User.self, byId: userId) {
switch $0 {
case .success(let user):
if let userWithWallet = user {
print(userWithWallet.name)
print(userWithWallet.status)
if let comments = userWithWallet.wallets {
for comment in comments {
print(comment.address ?? "")
}
}
} else {
print("User not found")
}
case .failure(let error):
print("User not found - \(error.localizedDescription)")
}
}
case .failure(let error):
print("Error retrieving users \(error)")
}
}
}) {
Text("READ Users")
}
}